With the distribution of users across different Operating Systems, there is a strong need for your software application work on wide range of Operating Systems and browsers. Otherwise there is a mere possibility that you will have only certain segment of customers with the operating system or browser that your software supports. Electron.NET Applications will help you build cross-platform application that run on wide range of Operating Systems and with chromium based rendering engine.
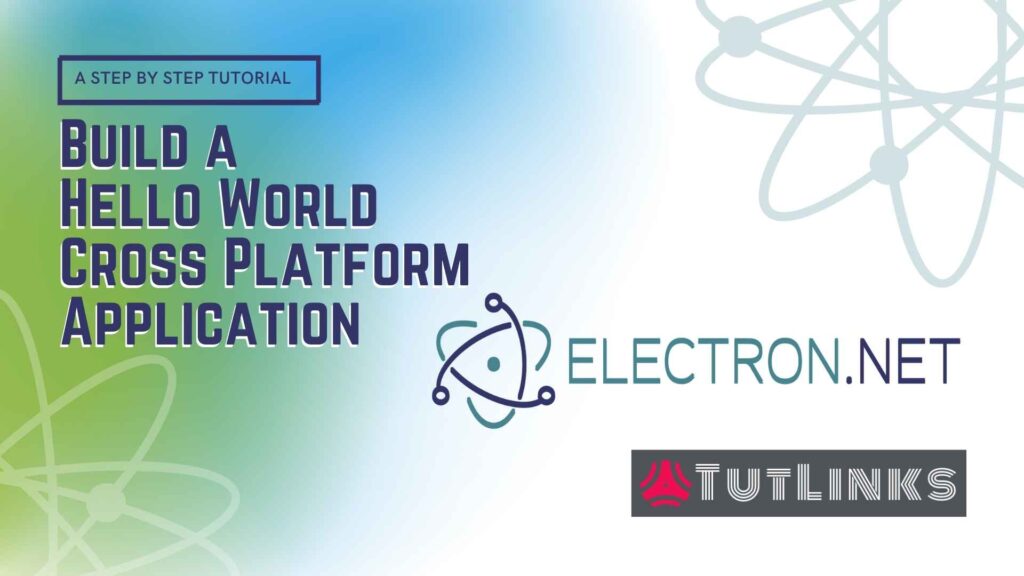
Table of Contents
- Need for Cross Platform Applications
- Setup Development Environment
- Create and Setup .NET Core project for Electron.NET Application
- Initialize Electron.NET Application
- Run the Electron.NET Application
- Build the Electron.NET Application
- Video Tutorial
Need for Cross Platform Applications
Imagine a situation that you have built a software application which runs on Mac OS and you make it to customers. You have made a decent customer base and your software gained popularity within short span of time. Now you look for expanding your customer base and plan to make your software application available to the users on Linux and Windows operating systems too. And no one ever really want to get in to a situation to start developing an existing software to support the new operating systems all from the scratch.
Electron is one such library written in Node JS and Chromium rendering engine. Electron offers the ability to build cross-platform desktop apps with JavaScript, HTML, and CSS.
It is highly likely that you might have used the software applications built using Electron. Skype, Slack, Visual Studio Code, Atom IDE, Discord, Signal are few are the most popular applications built with Electron.
Electron.NET Applications are typical ASP.NET Core applications wrapped inside the vanilla Electron framework. Electron.NET Application framework allows you to embed ASP.NET (Razor Pages, MVC) or Blazor applications to run on Electron as a host.
And do you know, you can build your First Hello World Electron.NET Application by following this step by step tutorial.
Full Source code is available here on github.
Setup Development Environment
Before we begin, we will setup the Development Environment. I am using Windows 10 OS. Yes, you can build a software application using Electron to target Windows, Linux and Mac OS at the same time.
- Ensure you have .NET 3.1 or latest runtime installed. I have .NET Core version 5.0.101 installed.
- For development and debugging of this application, I recommend you to install and use Visual Studio Code, an opensource IDE. VS Code is built with Electron!!
- Install the following VS Code extensions for
- CSharp
ms-dotnettools.csharp
and - dotnet
leo-labs.dotnet
- CSharp
Install ElectronNET.CLI available globally.
dotnet tool install ElectronNET.CLI -g
To check the version of ElectronNET.CLI run the command
electronize version
The output will be something like
ElectronNET.CLI Version: 9.31.2.0
Create and Setup .NET Core project for Electron.NET Application
Now lets start the development of our Hello World cross-platform application. We will host our Hello World cross-platform will run a ASP.NET Core 5 MVC application to run on Electron with the help of Nuget Package ElectronNET.API.
So lets start of by creating a project named tutlinks-electron-hello-world
using `dotnet` CLI as shown below.
mkdir tutlinks-electron-hello-world cd tutlinks-electron-hello-world dotnet new webapp code .
Add ElectronNET.API
package reference to the our project
dotnet add package ElectronNET.API
Notice the .csproj file updated with the package reference to ElectronNET.API
.
In Program.cs
file, import the package ElectronNET.API
by adding the following line before beginning of namespace.
// Program.cs using ElectronNET.API;
Now we want our webapp to create a Host using Electron framework. For that in the CreateHostBuilder
of Program.cs, add the following line that makes the webBuilder to use Electron. Also pass the args that are accepted by the CreateHostBuilder
to the UseElectron
method that is available on webBuilder of type IWebHostBuilder after we added using ElectronNET.API
// Program.cs public static IHostBuilder CreateHostBuilder(string[] args) => Host.CreateDefaultBuilder(args) .ConfigureWebHostDefaults(webBuilder => { webBuilder.UseElectron(args); //<-- Add this line webBuilder.UseStartup<Startup>(); });
Now, its time to modify Startup.cs
file. We will again add reference to ElectronNET.API in Startup.cs
// Startup.cs using ElectronNET.API;
Add the following line to the end of the Configure
method.
//Add this to the end of Configure method in Startup.cs if(HybridSupport.IsElectronActive) { CreateElectronWindow(); }
Now we will create a method named CreateElectronWindow
as follows.
// Startup.cs private async void CreateElectronWindow() { var window = await Electron.WindowManager.CreateWindowAsync(); window.OnMaximize += Window_OnMaximize; }
We also wireup an event and show a simple error box when our electron app is maximized.
Now lets define Window_OnMaximize
to show a simple error box
// Startup.cs private void Window_OnMaximize() { Electron.Dialog.ShowErrorBox("Maximized Event", "Hi, You just maximized your Electron App!"); }
Initialize Electron.NET Application
Now that we are setup with the basic environment and code changes necessary to spin up our web application in Electron, we will see how to run the application that opens in a Electron window.
The first thing we need to do is to initialize Electron for our web application. Doing so will generate a manifest with various properties like name of executable, image for splash screen, buld info to mention a few. To generate the Electron manifest, run the following command
electronize init
You will notice a manifest json file named electron.manifest.json
generated as the result of running the command electronize init
. You can modify the values of few properties of the manifest such as splashscreen
, author
and files
according to your needs.
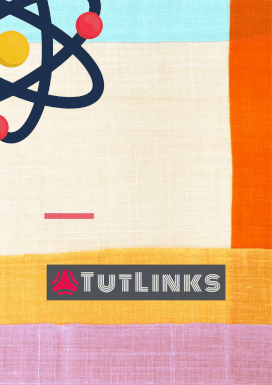
For example, if you want to show a fancy gif or image as a splash screen at the start up of your electron app, you can place the media in wwwroot/img
and refer it in electron.manifest.json
as follows
// electron.manifest.json // Comments doesn't really work in JSON. ;p. This is just for explainabilty. ... "splashscreen": { "imageFile": "/wwwroot/img/splash.gif" }, ...
Run the Electron.NET Application
As we successfully initialized our Electron App, its time to see how it looks like. So run the following command to see it up and running.
electronize start /watch
When you run this command for the very first time, dotnet will create the electron host at the location obj/Host
and will build and publishes all the dotnet assembiles of our application to the obj/Host/bin
location. It then invokes the electron application using node. As you might be aware that the typical node application starts by installing all the node modules based on the configuration in package.json and starts the electron host.
The /watch
argument also spins up the web browser and run the ASP.NET Core application in addition to running Electron App. This will be helpful during the development. But you can ignore the watch argument in case you don’t need it.
Build the Electron.NET Application
Once we are done with the development, we can build and generate the platform relevant executables by running the following command.
electronize build /target win electronize build /target osx electronize build /target linux
On Windows 10 OS, you need to run electronize build /target win
and the corresponding windows executable is generated with the name of the project at the bin\Desktop
location. The executable will generally be identified by the the format {YOUR PROJECTNAME} Setup 1.0.0.exe
.
Double click the executable to install the Hello World app on your PC. You can also find portable version of this application in the location bin\Desktop\win-unpacked
which you can archive and share it with your friends having Windows PC to boast your software application development skills.
You can also build the application to target Mac OS and Linux distros by running the following commands respectively.
electronize build /target osx electronize build /target linux